やりたいことは以下です
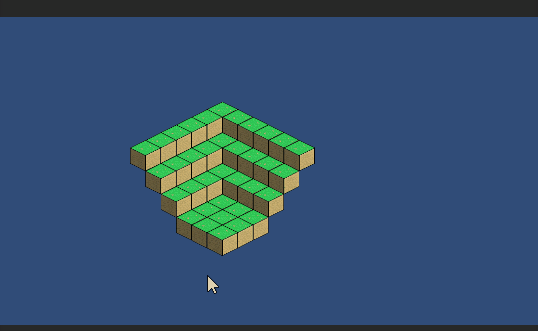
段差のあるTilemapでクリックしたセルの座標を正しく取得したいです!
どうすれば出来るか記載していきます!
(基本的にスクリプトで座標を取得することになります)
以下サイトの内容を参考にさせていただきました!

Clicking On Tiles With An Isometric Z As Y Tilemap?
I can't seem to register what tile is being clicked on with a z as y tilemap. Using WorldToCell and GetTile gives me the...
まずは段差のあるTilemapを作ろう!
最初に段差のあるTilemapを作成しないとなのですが
別で記載していますので以下をご確認ください!
段差のあるTilemapのセルの座標を取得するスクリプト全容
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Tilemaps;
public class TilemapInfo : MonoBehaviour
{
private int zMax;
[SerializeField]
private Tilemap tilemap;
[SerializeField]
private TileBase tile;
// Start is called before the first frame update
void Start()
{
zMax = tilemap.cellBounds.zMax;
Debug.Log("zMax = " + zMax);
}
// Update is called once per frame
void Update()
{
if (Input.GetMouseButtonDown(0))
{
GetTopCell();
}
}
// Z座標が高いセルの情報を取得します
public Vector3Int GetTopCell()
{
Vector2 mousePos = Camera.main.ScreenToWorldPoint(Input.mousePosition);
//Iterate through tile layers starting at the top
for (int i = zMax; i >= 0; i--)
{
Vector3 v = new Vector3(mousePos.x, mousePos.y, i);
//check cell
cell = tilemap.WorldToCell(v);
if (tilemap.HasTile(cell))
{
tilemap.SetTile(cell, tile);
break;
}
}
return cell;
}
}
ポイント1:全セルの最大のZ座標を取得する
最初のポイントは以下Start関数での処理です
void Start()
{
zMax = tilemap.cellBounds.zMax;
}
tilemap.cellBounds.zMaxでTilemap上の全てのセルの中で
最大のZ座標を取得しています
ポイント2:マウスクリックでGetTopCell()を呼び出す
続いてUpdate関数内でマウス左クリック時に
GetTopCell()を呼び出しています
このGetTopCell()が段差のセルの座標を取得する処理の本体です
void Update()
{
if (Input.GetMouseButtonDown(0))
{
GetTopCell();
}
}
ポイント3:マウスのセル座標を取得する
以下の部分でマウスのワールド座標(Vector2型)を取得しています
Vector2 mousePos = Camera.main.ScreenToWorldPoint(Input.mousePosition);
その後のfor文でStart時に取得したTilemapの最大のZ座標から0までループしています
for (int i = zMax; i >= 0; i--)
でfor文でループすることによって
以下でマウスのx座標,y座標は固定して
iでz座標を高い位置から低い位置に変更していって
Vector3の変数を設定しています
Vector3 v = new Vector3(mousePos.x, mousePos.y, i);
このVector3の変数から以下で更にTilemapのセル座標に変換しています
cell = tilemap.WorldToCell(v);
最後に以下でセル座標にセルが存在しているか確認し
存在していればそのセルを別のセルに置き換えています
if (tilemap.HasTile(cell))
{
tilemap.SetTile(cell, tile);
break;
}
解説おわり!
簡単に説明する形ですが
これでZ座標も考慮してマウスのクリックしたセル座標を
取得することができます!